By Raakesh T.
Java Archive (JAR) is a cross-platform archive file format used to compress and bundle multiple files (e.g. Java class files), metadata and resources into a single file with the .jar file extension. It is the preferred way for packaging Java applets or applications into a single archive, so that they may be downloaded by a browser with only a single request and response.
JAR files are built on the ZIP file format algorithm and are similar to UNIX’s tape archive format (TAR) and can be signed using digital signature to ensure authenticity.
Users can create or extract JAR files using the jar command that comes with a Java Development Kit (JDK). They can also use zip tools to do so; however when compressing, it’s important to note that the MANIFEST must first within the ZIP file order entries.
In the above example all the class files in the present directory will be bundled into the file called
To include a subdirectory in the JAR file:
The above example would bundle all the class files in the present directory and the all the contents in the subdirectory
If you have a preexisting manifest file and want to included specific name: value pairs, you can specify the with the
Be sure that any pre-existing manifest file that you use ends with a new line. Note that the order of the commands should be considered. For example using
Now the
When you sign the JAR file, the certificate containing the public key is created in the
A signature file with extension ".SF" is created in the
A signature block file with extension ".DSA" (Digital Signature Algorithm) is also created in
The signature related files are:
Note that if such files are located in
Before we sign the JAR file, we need to create private and public file required for encrypting and decrypting the JAR hash or digest value. JDK provides the
Following the above command you will be asked for the keystore password or to create one if the keystore does not yet exist and then, you will have to answer seven questions to record your identity. Then you will be asked to choose a password for the keys you just created.
To list the contents of
Now the key pair is generated which will be used to sign the JAR.
Note: Including the passwords on the command line is usually a bad idea – if you leave out the values then jarsigner will prompt you for them.
![]()
Download the JAR file and verify. You can extract the downloaded JAR file and manually parse the MANIFEST and .SF file data in the META-INF sub-directory.
![]()
The above option will not give any certificate information like Owner, Issuer or validity.
The JAR file can be verified using
If the JAR is unsigned, the response would be:
If the JAR is signed but the certificate is expired, the utility would give a warning that certificate is expired:
When you get the certificate expired message, you can read the certificate information using the
![]()
The above output would reveal the Owner, Issuer and Validity Information of the certificate.
It is also possible to read the certificate information from the .DSA file. To do so, extract the JAR file using a ZIP utility and then use openssl:
![]()
For more information - check out our whitepaper here:
References:
Java Archive (JAR) is a cross-platform archive file format used to compress and bundle multiple files (e.g. Java class files), metadata and resources into a single file with the .jar file extension. It is the preferred way for packaging Java applets or applications into a single archive, so that they may be downloaded by a browser with only a single request and response.
JAR files are built on the ZIP file format algorithm and are similar to UNIX’s tape archive format (TAR) and can be signed using digital signature to ensure authenticity.
Users can create or extract JAR files using the jar command that comes with a Java Development Kit (JDK). They can also use zip tools to do so; however when compressing, it’s important to note that the MANIFEST must first within the ZIP file order entries.
Creating JAR Files
To create a JAR file using the JDKjar
utility: C:\Java> jar cf Name.jar *.class
- c - Creates a new archive.
- f - Specifies the JAR file to be created.
In the above example all the class files in the present directory will be bundled into the file called
Name.jar
file. To include a subdirectory in the JAR file:
C:\Java> jar cf Name.jar *.class SubDirectory
The above example would bundle all the class files in the present directory and the all the contents in the subdirectory
SubDirectory
into a JAR file name Name.jar
.The MANIFEST
The manifest file,META-INF/MANIFEST.MF
, is automatically generated by the jar
tool and is always the first entry in the JAR file. The manifest file has meta-information about the archive is stored as name: value pairs. If you have a preexisting manifest file and want to included specific name: value pairs, you can specify the with the
m
option: C:\Java> jar cmf myManifestFile Name.jar *.class
Be sure that any pre-existing manifest file that you use ends with a new line. Note that the order of the commands should be considered. For example using
cfm
results in a different structured command from cmf
: C:\Java> jar cfm Name.jar myManifestFile *.class
Now the
MANIFEST.MF
has the following: Manifest-Version: 1.0
Created-By: 1.7.0_17 (Oracle Corporation)
Signing JAR Files
Thejarsigner
utility within the JDK can sign and verify JAR files. JAR files are signed using PKI (Public Key Infrastructure). PKI uses a public and private key pair - the private key should be kept with the owner privately and securely and the public key can made available publicly. Here the private is used to encrypt the file’s hash value and the public key will be used to decrypt the encrypted file’s hash value. Also to make the certificate genuine it has to be in the known Certificate Authority (CA) Chain. (The whole discussion on CA is out of scope).When you sign the JAR file, the certificate containing the public key is created in the
META-INF
directory. The digest (or hash) is computed for all files in the JAR and is also included in the manifest. Name: Name.class
SHA-256-Digest: (a 256-bit hash value for the file)
A signature file with extension ".SF" is created in the
META-INF
directory. The digest of each file is signed (or encrypted) using the signer's private key: Signature-Version: 1.0
SHA-256-Digest-Manifest-Main-Attributes: (base64 form of SHA-256 digest)
SHA-256-Digest-Manifest: (base64 form of SHA-256 digest)
Created-By: 1.7.0_17 (Oracle Corporation)
Name: Name.class
SHA-256-Digest: (base64 form of SHA-256 digest)
A signature block file with extension ".DSA" (Digital Signature Algorithm) is also created in
META-INF
directory. This file includes the digital signature for the JAR file, the digital certificate and the public key of the signer.The signature related files are:
META-INF/MANIFEST.MF
META-INF/*.SF
META-INF/*.DSA
META-INF/*.RSA
META-INF/SIG-*
Note that if such files are located in
META-INF
subdirectories, they are not considered signature-related. Before we sign the JAR file, we need to create private and public file required for encrypting and decrypting the JAR hash or digest value. JDK provides the
keytool
utility for managing public/private keys and digital certificates. The jarsigner
utility can be used for signing the JAR files. Generating keys
First you must create a pair of keys (private-public) which is used to sign the JAR and authenticate you. These keys can be generated using thekeytool
command. The generated keys are stored in a keystore
file. Each set of keys is associated with a unique name, known as its alias. To generate the keys: C:\Java> keytool -genkey -alias alias-name -keystore keystore-name
Following the above command you will be asked for the keystore password or to create one if the keystore does not yet exist and then, you will have to answer seven questions to record your identity. Then you will be asked to choose a password for the keys you just created.
To list the contents of
keystore
: C:\Java>keytool –keystore keystore-name –list
Now the key pair is generated which will be used to sign the JAR.
Signing
To sign the JAR file, use the following command C:\Java>jarsigner -keystore keystore-name -storepass keystore-password -keypass key-password jar-file alias-name
Note: Including the passwords on the command line is usually a bad idea – if you leave out the values then jarsigner will prompt you for them.
Check if a JAR is Signed
Your browser will automatically verify signed applets, if it can’t, it would throw a warning similar to the below: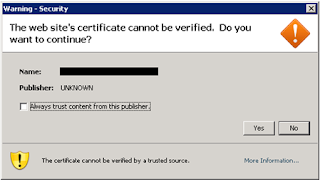
Download the JAR file and verify. You can extract the downloaded JAR file and manually parse the MANIFEST and .SF file data in the META-INF sub-directory.
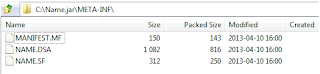
The above option will not give any certificate information like Owner, Issuer or validity.
The JAR file can be verified using
jarsigner
. The basic command to use for verifying a signed JAR file is: C:\Java>jarsigner -verify jar-file
If the JAR is unsigned, the response would be:
jar is unsigned. (signatures missing or not acessible)
If the JAR is signed but the certificate is expired, the utility would give a warning that certificate is expired:
jar verified.
Warning:
This jar contains entries whose signer certificate has expired.
This jar contains entries whose certificate chain is not validate
When you get the certificate expired message, you can read the certificate information using the
keytool
: C:\Java>keytool -list -printcert -jarfile jar-file.jar
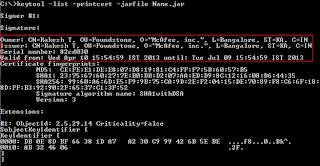
The above output would reveal the Owner, Issuer and Validity Information of the certificate.
It is also possible to read the certificate information from the .DSA file. To do so, extract the JAR file using a ZIP utility and then use openssl:
C:\>openssl pkcs7 -in signature-file.DSA -inform DER -print_certs -text
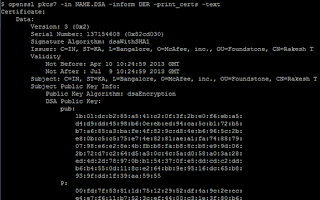
Conclusion
Signing the JAR with valid signature would help the users to identify malicious component publishers and modification of the components after publishing.For more information - check out our whitepaper here:
References: